Signals And Slots Across Threads Qt
- Signals And Slots Across Threads Qtc
- Qt Signal And Slots
- Qt Signal Slot Not Working
- Qt Signal Slot Example
Home > Articles > Programming > C/C++
␡- The goal of this course is to teach the student how to use design patterns in Qt 5 with C along with an understanding of issues specific to Qt 5. We will use a wide range of Qt technologies from Widgets, QML, sockets, server, threading, io, streams, processes, and the State Machine Framework.
- The code inside the Worker's slot would then execute in a separate thread. However, you are free to connect the Worker's slots to any signal, from any object, in any thread. It is safe to connect signals and slots across different threads, thanks to a mechanism called queued connections.
- Creating Threads
Signals & Slots, Signals and slots are made possible by Qt's meta-object system. To one signal, the slots will be executed one after the other, in the order they have valueChanged, and it has a slot which other objects can send signals to. The context object provides information about in which thread the receiver should be executed. Qt documentation states that signals and slots can be direct, queued and auto. It also stated that if object that owns slot 'lives' in a thread different from object that owns signal, emitting such signal will be like posting message - signal emit will return instantly and slot method will be called in target thread's event loop.
This chapter is from the book
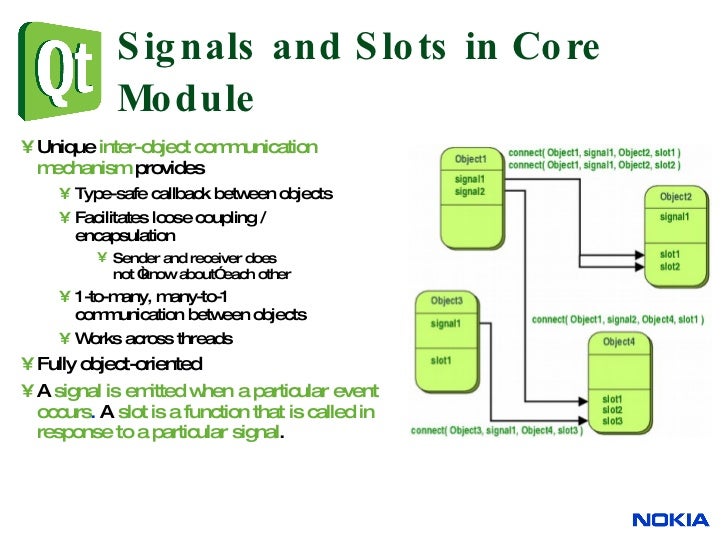
This chapter is from the book
This chapter is from the book
14. Multithreading
Signals And Slots Across Threads Qtc
- Creating Threads
- Synchronizing Threads
- Communicating with the Main Thread
- Using Qt's Classes in Secondary Threads
Conventional GUI applications have one thread of execution and perform one operation at a time. If the user invokes a time-consuming operation from the user interface, the interface typically freezes while the operation is in progress. Chapter 7 presents some solutions to this problem. Multithreading is another solution.
In a multithreaded application, the GUI runs in its own thread and additional processing takes place in one or more other threads. This results in applications that have responsive GUIs even during intensive processing. When runnning on a single processor, multithreaded applications may run slower than a single-threaded equivalent due to the overhead of having multiple threads. But on multiprocessor systems, which are becoming increasingly common, multithreaded applications can execute several threads simultaneously on different processors, resulting in better overall performance.
In this chapter, we will start by showing how to subclass QThread and how to use QMutex, QSemaphore, and QWaitCondition to synchronize threads. [*] Then we will see how to communicate with the main thread from secondary threads while the event loop is running. Finally, we round off with a review of which Qt classes can be used in secondary threads and which cannot.
Qt Signal And Slots
Multithreading is a large topic with many books devoted to the subject—for example, Threads Primer: A Guide to Multithreaded Programming by Bil Lewis and Daniel J. Berg (Prentice Hall, 1995) and Multithreaded, Parallel, and Distributed Programming by Gregory Andrews (Addison-Wesley, 2000). Here it is assumed that you already understand the fundamentals of multithreaded programming, so the focus is on explaining how to develop multithreaded Qt applications rather than on the subject of threading itself.
Creating Threads
Providing multiple threads in a Qt application is straightforward: We just subclass QThread and reimplement its run() function. To show how this works, we will start by reviewing the code for a very simple QThread subclass that repeatedly prints a given string on a console. The application's user interface is shown in Figure 14.1.
Figure 14.1 The Threads application
The Thread class is derived from QThread and reimplements the run() function. It provides two additional functions: setMessage() and stop().
The stopped variable is declared volatile because it is accessed from different threads and we want to be sure that it is freshly read every time it is needed. If we omitted the volatile keyword, the compiler might optimize access to the variable, possibly leading to incorrect results.


We set stopped to false in the constructor.
The run() function is called to start executing the thread. As long as the stopped variable is false, the function keeps printing the given message to the console. The thread terminates when control leaves the run() function.
The stop() function sets the stopped variable to true, thereby telling run() to stop printing text to the console. This function can be called from any thread at any time. For the purposes of this example, we assume that assignment to a bool is an atomic operation. This is a reasonable assumption, considering that a bool can have only two states. We will see later in this section how to use QMutex to guarantee that assigning to a variable is an atomic operation.
QThread provides a terminate() function that terminates the execution of a thread while it is still running. Using terminate() is not recommended, since it can stop the thread at any point and does not give the thread any chance to clean up after itself. It is always safer to use a stopped variable and a stop() function as we did here.
We will now see how to use the Thread class in a small Qt application that uses two threads, A and B, in addition to the main thread.
The ThreadDialog class declares two variables of type Thread and some buttons to provide a basic user interface.
In the constructor, we call setMessage() to make the first thread repeatedly print 'A's and the second thread 'B's.
When the user clicks the button for thread A, startOrStopThreadA() stops the thread if it was running and starts it otherwise. It also updates the button's text.
The code for startOrStopThreadB() is structurally identical.
If the user clicks Quit or closes the window, we stop any running threads and wait for them to finish (using QThread::wait()) before we call QCloseEvent::accept(). This ensures that the application exits in a clean state, although it doesn't really matter in this example.
If you run the application and click Start A, the console will be filled with 'A's. If you click Start B, it will now fill with alternating sequences of 'A's and 'B's. Click Stop A, and now it will print only 'B's.
Related Resources
- Book $31.99
Qt Signal Slot Not Working
- eBook (Watermarked) $25.59
Qt Signal Slot Example
- eBook (Watermarked) $28.79